How to use csv file for uploading Django model objects?
Introduction
Uploading Django model objects as a csv file is not a new concept. But in this post, we will talk about how to use a csv file and upload data into the database using Django’s built-in ORM. This method can be used when you want to avoid uploading Django model objects one by one. For example, if you want to let your user upload their products from csv file.
The pros of this method is that it minimizes time consumption for creating Django model objects. The user only uploads the csv file once, and then you have to read this file once or couple times but that’s it. No more network traffic or any other stuff with low priority. Why? Because reading a csv file is a very efficient thing to do on any system as it only requires reading files. Also we can use Django form validation to check whether the fields are valid or not. That will give you an extra benefit in terms of security because by having fewer endpoints, means that there are less possibilities for someone to mess with your code. The cons is it requires more work from the developer’s side because reading a csv file requires certain knowledge about how to split lines and how to parse them into proper data types. So if you prefer less network traffic, then go for it. If not, then depending on what your project needs, you can choose the method that best matches your project needs. To get started, first you need to have some Django model in your project. Let’s say we will be creating product categories.
Check your code
models.py
from Django.contrib.auth import get_user_model
from Django.db import models
from Django.urls import reverse
from master.models import TimeStamp
from product.managers import ProductManager
USER = get_user_model()
class TimeStamp(models.Model):
status = models.BooleanField(default=True)
created_on = models.DateTimeField(auto_now_add=True)
updated_on = models.DateTimeField(auto_now=True)
class Meta:
abstract = True
class CategoryModel(TimeStamp, models.Model):
name = models.CharField(max_length=48)
image = models.ImageField(
upload_to=”category/image/”, default=”default/category.png”
)
parent = models.ForeignKey(
“self”,
on_delete=models.SET_NULL,
null=True,
blank=True,
)
user = models.ForeignKey(USER, on_delete=models.CASCADE)
def __str__(self) -> str:
return self.name
def get_absolute_url(self):
return reverse(“product:category_detail”, kwargs={“pk”: self.pk})
forms.py
class FileUploadForm(forms.Form):
file = forms.FileField()
views.py
from csv import DictReader
from io import TextIOWrapper
from Django.contrib import messages
from Django.contrib.auth.mixins import LoginRequiredMixin
from Django.urls import reverse_lazy
from Django.views import generic as views
from .forms import FileUploadForm
class BulkCreateBaseView(LoginRequiredMixin, views.FormView):
template_name = “bulk_create/create.html”
form_class = FileUploadForm
success_url = reverse_lazy(“category_list”)
def form_valid(self, form):
file = form.cleaned_data.get(“file”)
file = TextIOWrapper(file, encoding=”utf-8″, newline=””)
rows = DictReader(file)
for row in rows:
obj = self.model.objects.filter(**row).first()
form = self.form_class(row, instance=obj)
if form.is_valid():
has_user = hasattr(form.instance.__class__, “user”)
if has_user:
form.instance.user = self.request.user
form.save()
else:
messages.error(self.request, form.errors)
return super().form_valid(form)
bulk_create.html
{% extends “base.html” %}
{% block content %}
Create Category From File
{% csrf_token %}
{{form.as_p}}
Create
{% endblock %}
Conclusion
We have discussed how to use csv file for uploading Django model objects. It is one of the effective way to handling the large dataset with the help of csv file. If you want to know more about Python, Django you can check out Learn Python Django training course in Kochi from the best Python full stack training institute in Kochi.
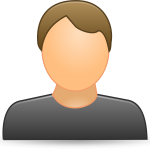