An Improved String Formatting Syntax: Python 3’s f-Strings

As of Python 3.6, f-strings are a new way to format strings. Not only are they more readable, more concise, and less prone to error than other ways of formatting, but they are also faster. Before Python 3.6, you had two ways of embedding Python expressions inside string literals for formatting: %-formatting operator and str.format().
f-Strings: An Improved Way to Format Strings in Python
f-strings (formatted string literals) are string literals that have an f
at the beginning and curly braces containing expressions that will be replaced with their respective values. The expressions are evaluated dynamically.
Simple Syntax
The syntax is similar to that of str.format() but less verbose. Look at how easily readable and understandable this is:
>>> name = "Eric" >>> age = 74 >>> f"Hello, {name}. You are {age}." 'Hello, Eric. You are 74.'
It would also be valid if we use a capital letter F
:
>>> F"Hello, {name}. You are {age}." 'Hello, Eric. You are 74.'
Arbitrary Expressions
As f-strings are evaluated at runtime, you can put any valid Python expressions in them. You could do something pretty straightforward, like this:
>>> f"{2 * 37}"
'74'
But you could also call functions. Here’s an example:
>>> def to_lowercase(input):
... return input.lower()
>>> name = "Eric Idle"
>>> f"{to_lowercase(name)} is funny."
'eric idle is funny.'
You also have the option of calling any method directly:
>>> f"{name.lower()} is funny."
'eric idle is funny.'
Speed
The f
in f-strings may as well stand
for “fast.”f-strings are faster than both %-formatting and
str.format()
. As you already saw,
f-strings are expressions evaluated dynamically rather than constant
values.
Here’s a speed comparison:
#Using % formatting
>>> import timeit
>>> timeit.timeit("""name = "Eric"
... age = 74
... '%s is %s.' % (name, age)""", number = 10000)
0.003324444866599663
#Using str.format()
>>> timeit.timeit("""name = "Eric"
... age = 74
... '{} is {}.'.format(name, age)""", number = 10000) 0.004242089427570761
#Using f-strings
>>> timeit.timeit("""name = "Eric"
... age = 74
... f'{name} is {age}.'""", number = 10000)
0.0024820892040722242
As you can see, f-strings come out on top.
You can still use the old ways of formatting strings, but with f-strings, you now have a more concise, readable, and convenient way that is both faster and less prone to error. Simplifying your life by using f-strings is a great reason to start using Python 3.6 if you haven’t already made the switch.
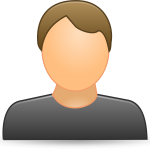