Comprehensions in Python
List Comprehension is one of the most widely used “Pythonic” syntaxes in Python, thus you’ve probably heard of it or used it before. It’s almost a requirement in most Python beginner’s lessons. You may have heard of or used Dictionary Comprehension before, but you may not be familiar with the set and generator.
List Comprehension:
This is without a doubt the most widely used in Python. It is very common and can be everywhere. The following is the basic syntax:
new_list=[<expression> for <item> in <iterable> if <condition>]
The condition is not required. Use it just when you need to apply a filter. So, to summarise: When you wish to keep the item the same, use expression = item. The condition will help to filter the iterable, resulting in fewer items being returned. Because condition is optional, the length of the iterable must be equal to my list if it is suppressed.
Dictionary Comprehension
A Dictionary Comprehension will generate a Dictionary.The basic syntax is as follows:
new_dict={<key>:<value> for <item> in <iterale> if <condition>}
It should be noted that the key and value can be expressions with or without item in this case.
Assume we have a list of dictionary objects containing the names, ages, and job titles of employees.
persons = [ { 'name': 'Alice', 'age': 30, 'title': 'Data Scientist' },
{ 'name': 'Bob', 'age': 35, 'title': 'Data Engineer' },
{ 'name': 'Chris', 'age': 33, 'title': 'Machine Learning Engineer' }]
The requirement is to get all the employees having their title contains the string “Data”. So, we can call them “data employees We also don’t care about the employees’ ages, thus we’d like to create a dictionary using the employees’ names as keys and their job titles as values. We can put all of this in one line and even show its readability using Dictionary Comprehension.
data_employees = {p['name']:p['title'] for p in persons if 'Data' in p['title']}
Set Comprehesion
Many people are unaware that Python has a Set Comprehension syntax. The only difference between a Set and a List Comprehension is that the former utilises curly braces instead of square brackets []. The syntax is as follows:
my_set = {<expression> for <item> in <iterable> if <condition>}
The difference between these two is that the Set Comprehension doesn’t have the key pair, but only one expression.
Data_employees_set={p[‘name’] for p in persons if ‘Data’ in p[‘title’]}
Keep in mind that the difference between a set and a list is that the items in a set cannot be repeated and not in order
Generator Comprehension
Last but not least, the majority of people are unaware of Generator Comprehension. The generator is a very special Python syntax; it is frequently generated from a function that uses the yield keyword rather than Python. The only difference between Generator Comprehension and List Comprehension is that the former uses parentheses.
my_gen = (<expression> for <item> in <iterable> if <condition>)
for example,
eg = (int(number/2) for number in my_list if number % 2 == 0)
rint(next(eg)) will result in first result. Likewise, repeat the print command till stop iteration exception occurs.
Comprehensions are considered as an important part in Python coding because of its ability to be concise and reduce significant code length. Python Django developer training in Kochi will help developing the coding skills from the beginner’s level. Choose a python full stack developer training in Kochi that will help you to reach the heights in your career.
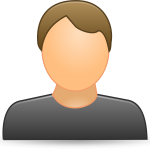