How to use Django Mixins?
Introduction
Mixins in Python are a useful construct that help you to abstract and share common functionality, while keeping your code clean. They provide a great way to organize your code and make it easier to maintain. But, if you understand the theory but were never able to apply them effectively, this post may be helpful. This blog post will walk you through an example of how Django Mixins work, show some things about how they’re used in practice, and give their use cases. It’s full of practical examples and advice for those who want to use mixins effectively! If you want to know more, please check out Python Django training in Kochi.
Mixing the best of Python with the power of design patterns is possible thanks to Django Mixins. Mixins are like a simple decorator in Python, except they’re not limited to just modifying functions. As the name suggests, they help you to mix the best of Django (web framework + ORM) with design patterns (factory, Singleton, and others).
Using mixins to encapsulate common functionality is a great way to provide idiomatic Django code.
But there are many circumstances when Django Mixins have subtle or not-so-subtle pattern-like qualities that make them powerful ingredients for designing reusable objects or design patterns. This post covers some examples where using Mixins helps prevent common problems in your code or allows you better structure of your codebase.
What are Django Mixins?
Django Mixins are Python abstract classes. They have a somewhat special property – the ability to be used as decorators.
Sometimes it’s useful to write some reusable code that you can use in many places of your project. This functionality can typically be encapsulated in a module (like utils.py), but sometimes it’s nicer if you want to implement it as part of an actual class within your codebase in such a way that it can also serve as a decorator or as a mixin, so that you can mix and match aspects and behaviors of one class with another. Django Mixin classes allow this type of design pattern.
While it’s possible to implement a mixin as a Python module, and then just apply it every time you want to use that functionality, using a Django Mixin class makes your code cleaner and easier to maintain. Here’s an example:
Let’s say you have SomeClass that looks something like this:
class SomeClass(object):
def __init__(self, x, y):
self.x = x
self.y = y
def do_stuff(self):
print(“SomeClass.do_stuff()”)
def do_stuff2(self):
print(“SomeClass.do_stuff2()”)
Other people in your team might like to use this codebase; you want to encourage them to do so, by making it easy for them to contribute to it and by keeping your codebase very well organized.
What you do is write a mixin:
class SomeMixin(object):
def __init__(self, some_class, other_mixin=None):
self.other = other_mixin or None
if other_mixin: # Other mixins work with this one too! self.other.do_stuff2()
self.other.do_stuff()
def do_stuff2(self):
print(“SomeMixin.do_stuff2()”)
def do_stuff(self):
print(“SomeMixin.do_stuff()”)
You are free to add other methods, of course. If you want to make this mixin more generic, e.g. make it work for any arbitrary class (not only SomeClass), you can do that too:
class SomeMixin(object):
def __init__(self, some_class):
self.some_class = some_class
# Mixin for classes, just like Django does
self.other = None # Holds references to other mixins
if hasattr(self.some_class, ‘do_stuff2’):
# Other mixins work with this one too!
self.other = self.some_class.do_stuff2
Now everyone on your team can use it this way:
from some_mixin import SomeMixin # Add other mixins here
from other_mixin import OtherMixin as OtherMixin # Add other mixins here
# Use the class as you would normally do!
SomeSomeClass = SomeClass(‘Hello’, OtherMixin())
print(SomeSomeClass.do_stuff())
print(OtherSomeClass.do_stuff())
You can also add other methods to the mixin, like this:
class SomeMixin(object):
def __init__(self, some_class):
# Mixin for classes, just like Django does
self.some_class = some_class
self.other = None # Holds references to other mixins
if hasattr(self.some_class, ‘do_stuff2’):
# do_something..
def do_stuff2(self):
pass
def do_stuff(self):
pass
An Intro to Design Patterns in Python Code Base with Django Mixins As you’ve seen above, a mixin is a class that provides additional functionality that can be mixed with some other class. This can be useful for providing common functionality across your codebase (rather than repeating yourself). As an example, a class that has additional functionality can be useful for setting up your database connections (like connecting to a database in order to show some information), or any other common functionality.
Conclusion
Django Mixins are a useful construct that help you to abstract and share common functionality. They provide a great way to organize your code and make it easier to maintain. This blog post will walk you through an example of how Django Mixins work in practice. Django Mixins are classes that provide additional functionality that can be mixed with other classes. Using a mixin makes your code cleaner and easier to maintain. An intro to Design Patterns in Python Code Base with Django Mixins is available on the Django website. If you want to learn about Django Fullstack development and best practices, you can check out Best Python full stack training institute in Kerala.
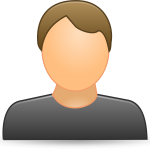