Python – Best Coding Practices
Introduction
PEP-8 is a set of coding guidelines for Python. PEP stands for Python Enhancement Proposal. Though not a law, it helps to establish best practices and shared conventions in the community when it comes to writing great code. This post will dive into the history of what motivated PEP-8, and how this document has been useful to the entirety of Python development. It discusses some of its potential benefits too, including preserving clarity and readability in your code-base over time. It emphasizes some of the principles that make PEP-8 so useful, and how they can be applied consistently in your Python projects.
Code review is vital to software development
Every developer knows that a code review is vital to software development practices. There are many reasons for this, from analysis to spotting potential bugs before they reach production. However, one of the most important facets of code reviews is reviewing existing practices and avoiding potential pitfalls that may harm the project’s success. Developers need to constantly be aware of the latest trends in programming tactics and keep up with what their peers are doing so they can avoid becoming obsolete. That’s not an easy task, though—and sometimes we start writing our code before we know what best practices are, leaving ourselves open to criticism on how our work could have been better done with past experiences. If you are new to python and want to know more, you can check out Python training in Kochi.
Popularity of Python
Python is a programming language that has gained popularity among a wide variety of developers in recent years. Not only does it make it easy to write code on the fly, but it’s also very good for quickly learning the basics of programming itself. That’s part of why we’re seeing more Python developers pop up these days—and we should be grateful for that, but also keep in mind that just because it’s an easy language to pick up doesn’t mean we can start writing bad code without learning best practices beforehand.
A small word about Program Maintainability
Maintainability is a big piece of the puzzle when working with code and defining best practices. Maintaining code is an ongoing process for a continuous number of reasons, from constantly improving existing programs to adding new features. Avoiding mistakes in the first place can cut down on the need to make human-error corrections later. It’s a skill set that is rarely given any attention, but just as important as any other aspect of a developer’s job.
This isn’t to say that every mistake will be caught right away or altered easily—but if we learn what we can ahead of time and avoid writing any bad code in the first place, it becomes much easier to catch existing mistakes and prevent them from becoming issues down the road.
What are some of the best practices?
So what are some of the best practices that you can learn from the Python community? We’ll be taking a look at a few of them over the coming weeks and breaking down why they contribute to making our jobs easier.
PEP 8, which is also known as “Docutils Style Guide”, outlines rules for writing clear and consistent documentation. It provides guidelines for four distinct types of documentation, being Bland Text, Examples, Sidebars, and Documentation. As a starting point for writing readable code, PEP 8 is an excellent guide to follow when writing Python code. It provides very specific guidelines for formatting and text file encoding (see pep-8.md).
Aside from the guidelines regarding coding style, there are a few more useful ways to make use of PEP 8 to improve the readability and maintainability of your code.
In many programming language communities, projects are run as library packages. These packages are often shared by many developers, and can even be downloaded into your local projects folder. As a result of this, developers will often put together many packages which contain functions that may seem unimportant when initially written but prove to be extremely useful later in the development process.
PEP 8 Pro Tip: Whether writing new code or reading old code, use __doc__ to get better documentation.
Since PEP 8 dictates methods for how to write readable source code, it can be a good idea to add the __doc__ method at the end of all your docstrings.
For example:
def factorial ( n ):
This will ensure that all instances of your function have a way to describe themselves in full detail, such as their arguments and their return values. It can also make it much easier for other developers when reading through the sources for your functions and will help them write assistants for more structured ways of documenting your code.
PEP 8 Pro Tip: Make your docstrings look great on GitHub.
Using Markdown with GitHub provides an easy way to add rich formatting to your documentation. It also makes it easier for GitHub to automatically render your docstrings in an attractive, easy-to-read layout.
PEP 8 Pro Tip: Use the #` delimiter in Python docstrings to create inline code snippets.
For inline code snippets, Python uses the #` delimiter. This makes it much easier to explain exactly what a particular function does or what parameters are needed when calling it.
This is particularly useful for helping other developers understand what your code is doing by giving them a chance to run it line by line.
PEP 8 Pro Tip: Don’t let trailing whitespace trip up your code.
Though most modern IDEs will keep you from making this mistake, there are still many times when extra whitespace can slip into your code. In cases like this, the PEP 8 standard dictates that we must use 4 spaces per indentation level, with no extra trailing whitespaces at the end of lines.
PEP 8 Pro Tip: Use underscore to separate two words.
Suppose we are declaring a variable called “hidden number”, we write it as hidden_number. This makes your code more readable.
PEP 8 Pro Tip: Use lowercase to declare variables, functions, and PascalCase for class names.
Conclusion
PEP-8 is a set of coding guidelines for Python. Python Enhancement Proposal-8 (PEP-8) is a set of coding guidelines for Python development. This post will dive into the history of what motivated this document, and how it has been useful to Python developers. It discusses some of its potential benefits too, including preserving clarity and readability in your codebase.
Python is an easy language to learn and use, but that doesn’t mean we can start writing bad code without learning best practices. PEP 8, also known as the “Docutils Style Guide”, outlines rules for writing clear and consistent documentation. Maintainability is a big piece of the puzzle when working with code and defining best practices. Hope you found it interesting to discover new things about Python. We haven’t discussed much, but these are some features that make Python incredible. If you want to know more about Python programming, check out the best python training institute in Kochi for beginners and advanced learners.
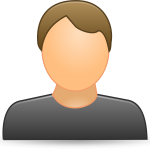