Some New Features in Python 3.8

Python 3.8 brings many new improvements to existing standard library packages and modules. math
in the standard library has some new functions. math.prod()
works similarly to the built-in sum()
, but for multiplicative products:
>>> import math
>>> math.prod((2, 8, 7, 7))
784
>>> 2 * 8 * 7 * 7
784
prod()
will be easier to use when you
already have the factors stored in an iterable.
Another new function ismath.isqrt()
. You can use isqrt()
to find the integer part of square roots.The square root of 9 is 3. You can see that isqrt()
returns an integer result, while math.sqrt()
always returns a float.The square root of 15 is almost 3.9. Note that isqrt()
truncates the answer down to the next integer, in the above case 3.
>>> import math
>>> math.isqrt(9)
3
>>> math.sqrt(9)
3.0
>>> math.isqrt(15)
3
>>> math.sqrt(15)
3.872983346207417
Finally, you can now more easily and efficiently work with n-dimensional points and vectors in the standard library. You can find the distance between two points with math.dist()
, and the length of a vector with math.hypot()
>>> import math
>>> point_1 = (16, 25, 20)
>>> point_2 = (8, 15, 14)
>>> math.dist(point_1, point_2)
14.142135623730951
>>> math.hypot(*point_1)
35.79106033634656
>>> math.hypot(*point_2)
22.02271554554524
This makes it easier to work with points and vectors using the standard library math.
The statistics
module also has some new functions:
statistics.fmean()
calculates the mean of floating point numbers.statistics.geometric_mean()
calculates the geometric mean offloat
numbers.statistics.multimode()
finds the most frequently occurring values in a sequence.statistics.quantiles()
calculates cut points for dividing data into n continuous intervals with equal probability.
>>> import statistics
>>> data = [9, 3, 2, 1, 1, 2, 7, 9]
>>> statistics.fmean(data)
4.25
>>> statistics.geometric_mean(data)
3.013668912157617
>>> statistics.multimode(data)
[9, 2, 1]
>>> statistics.quantiles(data, n=4)
[1.25, 2.5, 8.5]
In Python 3.8, there is a new statistics.NormalDist
class that makes it more convenient to work with the Gaussian normal distribution
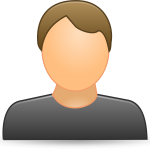